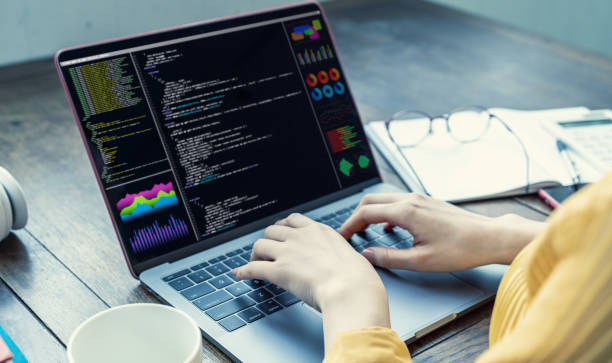
Java stands as one of the most popular and versatile programming languages in the world of software engineering. Its robustness, platform independence, and extensive ecosystem make it a top choice for developing a wide range of applications, from mobile apps to enterprise-level systems. Understanding the fundamentals of Java is crucial for anyone aspiring to become a proficient software engineer. In this article, we delve into the essential building blocks of Java and how they form the foundation of software engineering.
Introduction to Java
Java, developed by James Gosling and his team at Sun Microsystems in the mid-1990s, quickly gained traction due to its simplicity, reliability, and security features. It was designed to be platform-independent, allowing developers to write code once and run it anywhere, a concept encapsulated in the slogan “Write Once, Run Anywhere” (WORA).
Variables and Data Types
Variables serve as containers for storing data in a Java program. Understanding the different data types available in Java is essential for effective programming:
- Primitive Data Types: These are the basic data types provided by Java, including integers (int), floating-point numbers (float, double), characters (char), boolean values (boolean), etc.
- Reference Data Types: These include objects, arrays, and strings. Unlike primitive data types, reference data types store references to the actual data rather than the data itself.
Control Flow Statements
Control flow statements determine the execution order of code within a Java program. These statements allow developers to control the flow of execution based on certain conditions:
- Conditional Statements (if, else if, else): These statements execute different blocks of code based on the evaluation of conditions.
- Looping Statements (for, while, do-while): These statements allow the execution of a block of code repeatedly based on a condition.
- Switch Statement: It provides a convenient way to select one of many code blocks to be executed.
Methods and Functions
Methods (or functions) are blocks of code that perform a specific task and can be called from other parts of the program. Understanding how to define and use methods is crucial for organizing and modularizing code with Java software development services:
- Method Declaration: A method is declared with a return type, name, parameters (if any), and a body containing the code to be executed.
- Method Invocation: Methods are called or invoked using their name along with any required arguments.
- Return Values: Methods can optionally return a value to the caller using the return statement.
Object-Oriented Programming (OOP) Concepts
Java is an object-oriented programming language, which means it follows the principles of encapsulation, inheritance, polymorphism, and abstraction:
- Classes and Objects: Classes serve as blueprints for creating objects, which are instances of a class. Objects encapsulate data and behaviour.
- Inheritance: It allows a class to inherit properties and behaviour from another class, promoting code reusability.
- Polymorphism: It enables objects to be treated as instances of their parent class, facilitating flexibility and extensibility.
- Abstraction: Abstraction hides the complex implementation details of a class, exposing only essential features.
Exception Handling
Exception handling is a crucial aspect of Java programming for dealing with unexpected situations or errors that may occur during runtime:
- Try-Catch Blocks: These blocks allow catching exceptions and handling them gracefully without crashing the program.
- Throwing Exceptions: Developers can manually throw exceptions using the throw keyword to signal exceptional conditions.
- Finally Block: The final block ensures that certain code executes whether an exception is thrown or not, making it useful for cleanup operations.
Input and Output Operations
Java provides various classes and methods for performing input and output operations, allowing interaction with users and external resources:
- Reading Input: The Scanner class is commonly used for reading input from the keyboard.
- Writing Output: The System.out.println() method is used to display output to the console.
- File I/O: Classes like FileReader, FileWriter, BufferedReader, and BufferedWriter facilitate reading from and writing to files.
Arrays and Collections
Arrays and collections are used for storing and manipulating multiple values in Java:
- Arrays: Arrays are fixed-size containers that hold elements of the same data type. They offer efficient random access to elements.
- Collections Framework: The Collections framework provides interfaces and classes for working with collections of objects, such as lists, sets, and maps. It offers dynamic resizing and additional functionality compared to arrays.
Concurrency
Concurrency is the ability of a program to execute multiple tasks simultaneously. Java provides robust support for concurrency through its built-in features:
- Threads: Threads are lightweight processes that run concurrently within a Java program. They allow the execution of multiple tasks simultaneously.
- Synchronization: Synchronization mechanisms such as locks and synchronized blocks ensure thread-safe access to shared resources, preventing data corruption and race conditions.
- Concurrency Utilities: Java’s Concurrency Utilities framework provides high-level constructs like Executors, Callable, and Future for managing concurrent tasks efficiently.
Conclusion
Mastering the fundamentals of Java lays a solid groundwork for aspiring software engineers. By understanding variables, control flow, methods, object-oriented concepts, exception handling, I/O operations, arrays, collections, and concurrency, developers can build robust and efficient applications. Continuously honing these skills and exploring advanced topics will empower developers to tackle complex software engineering challenges with confidence.